10 min read
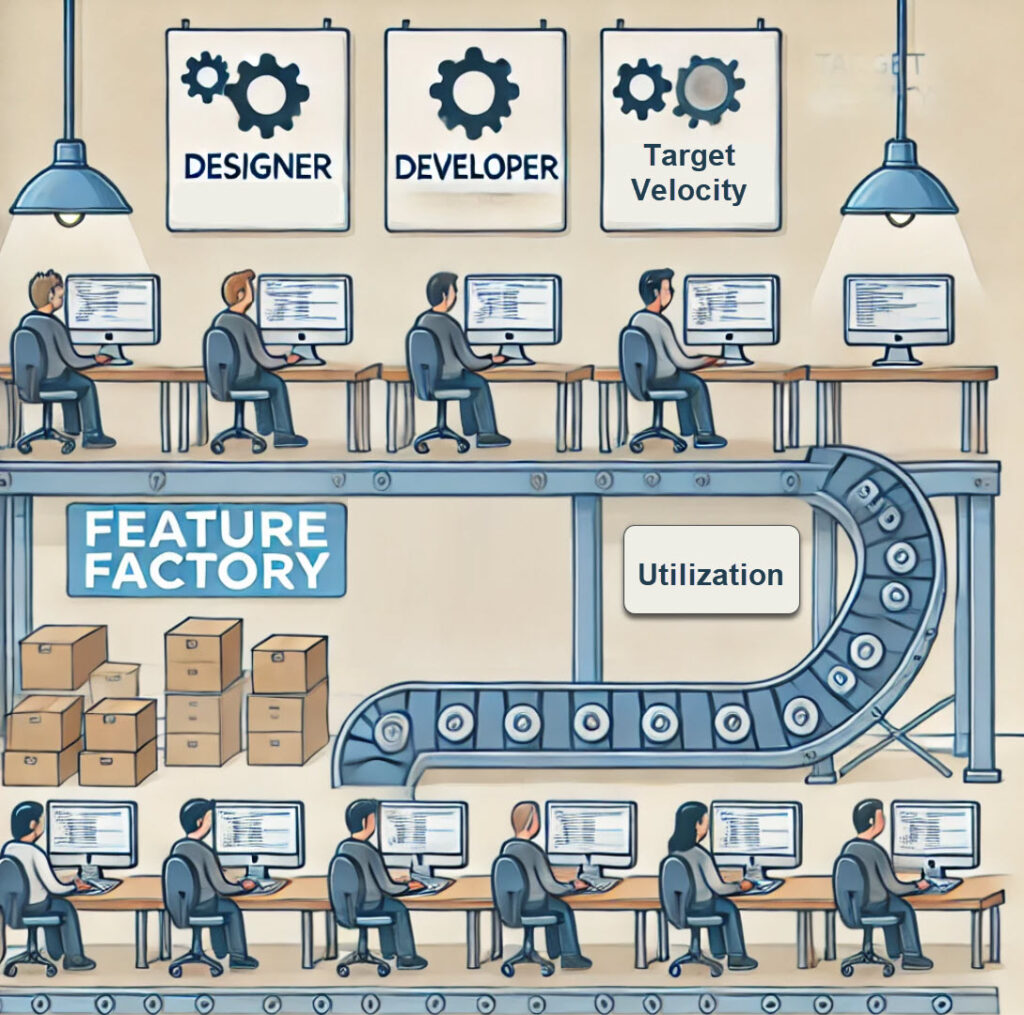
This article is the final part of a series on linking software engineering to business success. If you missed the earlier articles, start here.
This Article at a Glance
This article explores how engineering teams can escape the “build trap” and move beyond a feature factory mindset by focusing on outcomes instead of outputs. It highlights the pitfalls of the build trap, the value of starting with outcomes, fostering accountability and alignment, and ensuring successful delivery. Aimed at technology leaders, Product Managers, and teams, it challenges them to adopt a mindset centered on meaningful outcomes—driving engagement, alignment, and impactful business results.
From Speed to Meaningful Value
Picture this: you’re leading a team of talented developers, launching features rapidly, but customer satisfaction isn’t improving, and the business impact is unclear. This happens when teams focus too much on output, a common issue in traditional project-based management. Switching to a product operating model and applying Value Stream Management can break this cycle. These approaches focus on outcomes instead of outputs, ensuring every effort is tied to measurable business value.
As a senior technology leader, I’ve been there. Early in my career, I focused on operational efficiency and rapid feedback, confident that speed and volume would drive success. But I learned the hard way: speed alone doesn’t guarantee value.
This realization highlighted a critical gap—the need for realization. Efficiently delivering work is only part of the puzzle; the priority must be ensuring that every effort contributes meaningful outcomes for both customers and the business.
To close this gap, I began asking:
- What outcomes do we expect, how will we measure success, and what can we learn from the results?
By adopting value stream management (VSM) and objectives and key results (OKRs), we created a clear link between work and impact. VSM revealed how work flows through teams and where value is created, while OKRs provided a framework for aligning team goals with organizational priorities.
Escaping the build trap isn’t just about faster delivery—it’s about rethinking success. When efforts are tied to measurable results, teams and leaders work with clarity, purpose, and trust, transforming delivery into meaningful value for the business and its customers.
Defining Outcomes at Every Level
Anticipated Outcomes for Epics
In Agile software development, an initiative is a large, highest-level body of work representing a significant feature or functionality too extensive for a single sprint. Initiatives usually have Epics defined to support a piece of the Initiative. Defining anticipated outcomes for each Initiative or epic clarifies the value being delivered, the behavior being changed, and how success will be measured. This approach helps teams see their work’s purpose and anticipated impact and how it aligns with organizational goals, as well as create team-level OKRs within their focus areas.
Questions to define Initiative or Epic level outcomes:
- What problem are we solving, what behavior are we changing, or what opportunity are we addressing?
- What results do we expect, and how will we measure them?
- What metrics will define success?
- How will we close the loop by learning from actual outcomes?
Example: An initiative aimed at improving onboarding might define success metrics like:
- Increasing customer retention by 10% within 30 days.
- Reducing onboarding-related support tickets by 15%.
- Receiving positive feedback from customer satisfaction surveys.
By defining these outcomes, teams can create Key Results that align with broader organizational objectives, ensuring their efforts directly support larger goals. These key results can become the objectives of supporting epics.
Outcomes-Driven Iterations
Initiatives or Epics provide the overarching product change or improvement, while sprints should center on value-driven outcomes, not merely task completion. Traditionally, sprints have prioritized finishing backlog tasks. However, by integrating Value Stream Management with a product operating model, the focus shifts toward delivering meaningful value. Instead of measuring success by completed tasks, sprints are now about achieving impactful results aligned with value streams. This approach ensures that every iteration targets tangible customer and business results.
Teams should define sprint goals based on outcomes, not tasks.
Example: “Enhance system performance by improving response times by 5%” (outcome) versus “Complete three refactoring tickets” (task).
This mindset shifts how teams view their work:
- Collaboration over individual output: Team members who finish early should check if the sprint goal has been met and help others achieve it.
- Focus on shared success: Success is measured by achieving the outcome, not individual task completion.
When iteration goals align with epic outcomes, teams focus on delivering meaningful value at every level of work.
OKRs, focus on organization alignment
OKRs are essential for helping teams break free from the build trap by connecting their efforts to impactful outcomes. By focusing on work (features, technical debt, defects, and risks) within the team’s scope or control, OKRs align the team’s work with customer needs and organizational objectives. This alignment transforms sprints, epics, and initiatives from mere tasks into measurable milestones that drive meaningful business results.
OKRs bridge the gap between your team’s efforts and the outcomes that truly matter, encouraging a focus on value rather than speed. This mindset shift fosters intentional work and delivers impactful results.
To develop a team-level OKR from a parent key result, you can use a method known as explicit alignment or cascading. This process transforms a higher-level key result into a focused objective for your team, ensuring clear alignment and purpose. Here’s an effective way to approach it:
- Define your desired outcome and ensure it aligns with a relevant Parent Key Result:
Begin by reviewing the overarching OKRs at the company or department level. Identify a key result that aligns with your team’s responsibilities and can be directly influenced within your team’s scope. - Transform the Key Result into an Objective:
Reframe the parent key result as a clear objective for your team (the expected outcome). This objective will serve as the central focus of their efforts. - Develop Supporting Key Results:
Create 3-5 key results to help your team achieve this new objective. These should be specific, measurable, and aligned with the overall goal or outcome. - Ensure Alignment:
Make sure your team’s OKRs align with and support the higher-level objective they are based on. Set clear targets for each key result by defining what success looks like for your team about the parent key result. This will help keep your team focused and guide their efforts toward achieving the desired outcome.
Note: Escaping the build trap requires more than focusing on outcomes—it demands a structural shift to a product operating model. By aligning teams with specific products and value streams, organizations create an environment where teams are not just delivering features but owning the evolution of outcomes over time. This model encourages accountability and allows teams to iteratively refine their work, fostering alignment with customer needs and organizational goals. It also reduces handoffs and inefficiencies, enabling teams to focus on continuous delivery and improvement.
Accountability and Team Alignment
The Role of Product Managers
A strong product team is defined by the trust and partnership between engineers and their Product Manager—someone engineers would confidently “go to bat for.” To build this trust, Product Managers must prioritize technical debt, risks, and defects alongside new features, acknowledging their critical role in delivering a high-quality product.
Product Managers must also actively engage as part of the team. Their role involves:
- Collaborating on priorities.
- Participating in discussions.
- Supporting the shared goal of delivering value to customers.
Ownership
Every outcome or team-level OKR needs a clear owner—someone who is accountable for defining, prioritizing, and ensuring its achievement. In Agile teams, this often means Product Managers own the outcomes tied to customer-facing features, while technical leads take responsibility for outcomes related to technical debt, scalability, or system performance. For example:
- A Product Manager might own the outcome of increasing user retention by 10% through a new onboarding feature, ensuring the team understands the goal and tracks metrics like retention or onboarding completion rates.
- A Technical Lead might own the outcome of reducing downtime by 15% by addressing key infrastructure improvements, ensuring technical debt is addressed in a way that aligns with broader organizational goals.
Ownership ensures clarity and accountability, preventing outcomes from falling through the cracks or becoming vague aspirations. The owner is also responsible for closing the loop—documenting the actual outcomes, sharing them with stakeholders, and reflecting on whether the actual outcomes were achieved.
Prioritizing by Anticipated Outcome or Impact
There’s always more work than resources. Your team is limited by the people you There’s always more work than resources. Your team is limited by the people you have and the time available. Capacity limits make it essential to prioritize based on outcomes. When new tasks arise, check if they align with your current objectives or OKRs. Don’t hesitate to push back if they don’t contribute to key objectives. Ask yourself:
- Does this work align with our top priorities? If not, why should it take precedence?
- What will we remove or deprioritize to maintain focus if we take on this work?
- How does this impact our ability to achieve current targets and objectives?
When new work aligns with your objectives or introduces a higher-priority goal, something else must be deprioritized to make room. Collaborate as a team to identify and remove the lowest-priority work and communicate the updated goals or OKRs.
Prioritizing work based on anticipated return on investment (ROI) and anticipated outcomes ensures that engineering efforts focus on initiatives with the greatest potential for business impact. This approach balances short-term needs with long-term value creation, guiding teams to deliver meaningful results.
By recognizing capacity constraints and ensuring all stakeholders understand new requests’ significance and expected impact, teams can align their efforts with expected outcomes and ROI. This approach fosters collaboration, accountability, and a shared commitment to purposeful progress among Product Managers, engineers, and stakeholders.
Aligning Incentives
Teams must recognize the tensions created by differing role incentives:
- Product Managers are often rewarded for growth and feature delivery.
- Engineers focus on quality, performance, and resilience.
These priorities can clash, but alignment is achievable when both roles focus on shared outcomes. Product Managers who treat technical debt, quality, and security as essential aspects of the product—not competing concerns—foster trust and collaboration within their teams.
Great engineers go beyond technical skills—they understand the product and its goals. They ask thoughtful questions to ensure their work meets customer and business needs. This understanding helps them propose pragmatic solutions, such as quickly delivering 80% of the value while planning to address the remaining 20% later. By balancing speed and quality, engineers with a product mindset help their teams avoid becoming a “feature factory” that builds without considering impact or value.
When teams hold all members—particularly Product Managers responsible for features—accountable for defining outcomes, measuring success, and closing the feedback loop, they achieve greater clarity and alignment. This accountability drives purposeful work, encourages shared ownership, and links meaningful outcomes directly to business goals.es, measuring success, and closing the feedback loop, they achieve greater clarity and alignment. This accountability drives purposeful work, encourages shared ownership, and links meaningful outcomes directly to business goals.
Closing the Loop
I am a huge fan of “closing the loop” or sharing the outcome of the team’s efforts with the team and organization. Success isn’t just about finishing tasks; it’s about creating meaningful results. Instead of focusing on whether something is “done,” the impact measures real success: Did our work lead to an apparent, positive, and measurable change in customer behavior? Closing the loop is a key part of this process, and Product Managers and technical leads need to prioritize it to achieve lasting outcomes.
Although it may take weeks, months, or longer to receive the data or feedback, documenting the actual outcomes—whether in the Initiative, Epic, or both—is essential to show how the team performed. Metrics like customer engagement, retention, or efficiency gains help determine if the work delivered its intended value. For instance, if a new feature was meant to reduce churn, tracking churn rate, customer feedback, or increased product usage can confirm if the goal was met. Without this step, teams lose an essential chance to learn from their work. It shows how well the team met its objectives, helps improve future decisions, and ensures continuous growth.
Incorporating “closing the loop” into workflows helps Product Managers and technical leads gain actionable insights from every project. It ensures teams receive feedback on their work, promoting accountability, alignment, and constructive improvement. This approach keeps the focus on achieving clear outcomes and measurable success.
From Delivery to Realization
We’re enhancing team performance and operational efficiency by adopting a Product Operating Model and incorporating Value Stream Management (VSM) and Objectives and Key Results (OKRs) into our processes. Unlike projects with a defined end date, products follow a lifecycle—we’re not finished until the product is retired. This approach allows us to align teams to products and continuously deliver updates and improvements throughout a product’s lifecycle.
Value Stream Management (VSM) enables us to visualize the entire flow of value delivery, helping us identify and address bottlenecks and inefficiencies. Meanwhile, OKRs provide a structured framework for setting and tracking team goals, ensuring alignment with the organization’s broader strategic objectives. Together, these tools drive focus, clarity, and measurable progress in delivering value to our customers.
Integrating these practices is transforming our culture. Success is no longer measured by speed or output alone—it’s defined by the value delivered. These practices empower teams to recognize their impact, align with shared goals, and clearly demonstrate how their efforts drive business performance.
Lead With Outcomes
The time for teams to focus on both delivery and realization is now.
Steps to transform your teams:
- Define outcomes first: Start every epic, sprint, and initiative with clear, measurable outcomes.
- Hold teams accountable: Ensure product managers and engineers align with outcomes, not just output.
- Close the loop: Measure actual results, share insights with the team, and learn from every outcome through retrospectives.
When teams align on outcomes and focus on delivering impactful value, they move beyond simply following orders. They gain clarity on their purpose and unlock their full potential. We can create products that provide meaningful customer value while demonstrating clear contributions to organizational success.
Let’s lead with expected outcomes and break free from the build trap—together.
Series Summary
Related Posts: Metrics and Team Efficiency
- Navigating the Digital Product Workflow Metrics Landscape: From DORA to Comprehensive Value Stream Management Platform Solutions, August 31, 2024
- A Balanced Approach to Agile Metrics: Empowering Teams and Mitigating Risks, March 02, 2024.
- Mitigating Metric Misuse: Preventing the Misuse of Metrics and Prioritizing Outcomes Over Outputs, June 21, 2023.
- Developer Experience: The Power of Sentiment Metrics in Building a TeamX Culture, June 18, 2023.
- Outcome Metrics and the Difficulty of Reporting on Value, February 18, 2023.
- Maximizing Technology Team Performance: Insights from a CEO Conversation, February 15, 2023.
- Finally, Metrics That Help: Boosting Productivity Through Improved Team Experience, Flow, and Bottlenecks, December 29, 2022.
Poking Holes
I invite your perspective on my posts. What are your thoughts?.
Let’s talk: [email protected]